# Base Conversion
This article will go over how to convert between decimal numbers to binary, hex, as well as any other base number.
Now, to get our terminology straight:
| Term | Explanation |
| ---- | ----------- |
| Base | A `base` represents how many numbers can be used to count with. Base 2 uses 2 numbers, 0 and 1, base 4 would use 4 numbers, 0, 1, 2, and 3. Commonly numbers above 9 are presented using the latin alphabet, with 10 being *A*, 11 being *B*, etc. |
| Integer | An `Integer` presents a whole number. 1, 2, 3, 5, 10, 30000 are all `integers`. 12.5 is *not* an `integer` |
| Floor Value | A `Floor Value` is an integer resulting from rounding down a number. `2.5`, `2.7`, `2.1`, would all have a `Floor value` of `2` |
## Convert from decimal to any other base
Given a number in base 10, convert it to base `N`.
The solution is to divide the number by the base and store the remainder. The remainder will be a digit of the new base. The number's floor value is then divided by the base again and the process is repeated until the number is zero.
If the base is higher than the digits that are stored in a list, the result is already in front of you.Then reversed to get the correct order.
The guide will use examples of binary (base 2) and hexadecimal (base 16), but it will work with any base.
Example:
```
number = 10, base = 2
10 / 2 = 5 remainder 0
5 / 2 = 2 remainder 1
2 / 2 = 1 remainder 0
1 / 2 = 0 remainder 1
The base 2 value of 10 would be 1010
```
This conversion works with base 10 to ANY base.
Here's with base 16:
```
number = 10, base = 16. Numbers 10-15 are represented by A-F
10 / 16 = 0 remainder 10 (A)
The base 16 value of 10 would be A
```
As the value 10 is already under the base value of 16, the value is directly convertable to hex. Let's use some other values over the baes value of 16.
```
number = 27, base = 16
27 / 16 = 1 remainder 11 (B)
1 / 16 = 0 remainder 1 (1)
The base 16 value of 27 would be 1B
```
```
number = 255, base = 16
255 / 16 = 15 remainder 15 (F)
15 / 16 = 0 remainder 15 (F)
The base 16 value of 255 would be FF
```
## Convert back to base 10
The conversion back to base 10 is done by multiplying the digit by the base to the power of the position of the digit. The sum of all these values is the base 10 value.
Now that was a lot of words, so in short, `c * b ^ i` \-\- where `c` is the digit, `b` is the base and `i` is the position of the digit from right to left, starting at 0.
Below is a table to visualize this a bit better.
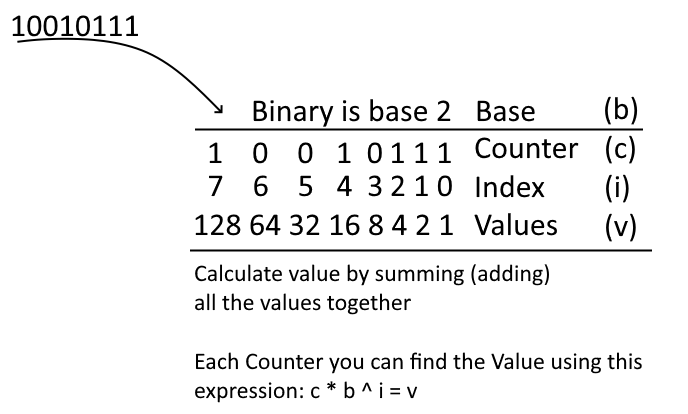
Example:
```
number = 1010, base = 2
1 * 2 ^ 3 = 8
0 * 2 ^ 2 = 0
1 * 2 ^ 1 = 2
0 * 2 ^ 0 = 0
8 + 0 + 2 + 0 = 10
The base 10 value of 1010 would be 10
```
Same goes for base 16:
```ts
number = 1B, base = 16
1 * 16 ^ 1 = 16
11 * 16 ^ 0 = 11
16 + 11 = 27
The base 10 value of 1B would be 27
```
## Cheatsheet for value representation
| Base | Name | Values |
| ---- | ---- | ------ |
| 2 | Binary | 0 1 |
| 4 | Quaternary | 0 1 2 3 |
| 8 | Octal | 0 1 2 3 4 5 6 7 |
| 10 | Decimal | 0 1 2 3 4 5 6 7 8 9 |
| 16 | Hexadecimal | 0 1 2 3 4 5 6 7 8 9 A B C D E F |